Motor
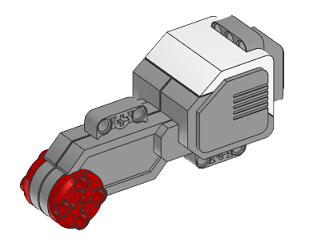
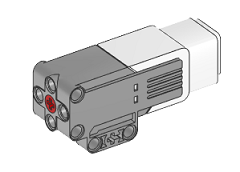
-
class
devices.
Motor
(ev3handle, layer=1, port='A')[source] The class to represent the EV3 motor.
Set up a motor on output port
'B'
>>> from pyev3.brick import LegoEV3 >>> from pyev3.devices import Motor >>> myev3 = LegoEV3(commtype='usb') >>> mymotor = Motor(myev3, port='B')
Set up a motor on output port
'A'
of the first brick and port'B'
of the second brick>>> mymotor1 = Motor(myev3, layer=1, port='A') >>> mymotor2 = Motor(myev3, layer=2, port='B')
- Parameters
ev3handle (object) –
LegoEV3
instance representing the EV3 brick.layer (int) – The layer of the brick
1
or2
in a daisy-chain configuration.port (str) – The brick output port connected to the motor. Possible values are
'A'
,'B'
,'C'
, or'D'
.
Note
1. If EV3 bricks are connected in a daisy-chain configuration, set the input parameter layer to the appropriate number.
-
stop
(brake='off')[source] Stop the EV3 motor.
- Parameters
brake (str) – The brake option of the motor
'on'
or'off'
. Can be used to hold the motor position.
>>> mymotor.stop(brake='on')
-
property
angle
Contains the angular position of the motor in degrees (read only).
-
property
output
Contains the motor output level as a percentage. Values can be between
-100
or100
(read/write).
-
property
outputmode
Contains the motor output mode. It can be either
'speed'
or'power'
(read/write). The motor has to be stopped before switching modes.