LegoEV3
The LEGO® MINDSTORMS® EV3 brick is at the core of the pyev3 package. Once the brick is powered up, you can use a PC to communicte with it in two ways:
USB (fastest)
requires a USB cable (that comes with the set)
very repeatable command/reply round-trip times of approx. 5 ms
WiFi (free to move around!)
requires a WiFi dongle (purchased separately)
not very repeatable command/reply round-trip times between 10 to 20 ms
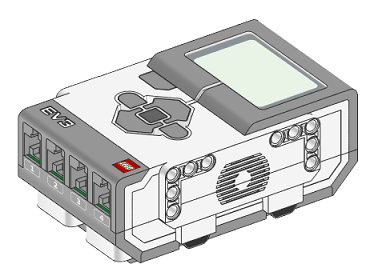
-
class
brick.
LegoEV3
(commtype='usb', IPaddress=None, deviceID=None)[source] The class to represent the LEGO EV3 brick. You can use LegoEV3 to interact with the EV3 brick.
Set up USB connection between host and EV3 brick.
>>> from pyev3.brick import LegoEV3 >>> myev3 = LegoEV3(commtype='usb') >>> myev3.display_info() >>> myev3.close()
Set up WiFi connection between host and EV3 brick
>>> myev3 = LegoEV3(commtype='wifi', IPaddress='192.168.0.19', deviceID='001653470e58') >>> myev3.display_info() >>> myev3.close()
- Parameters
commtype (str) – The type of communication with the brick.
'usb'
or'wifi'
.IPaddress (str) – The IP address assigned to the EV3 brick.
deviceID (str) – The individual device ID of the EV3 brick. Connect to the brick using commtype=’usb’ and use the display_info() method to retrieve the ID of the brick.
Note
Always use the close() method before opening a new connection.
Try a USB connection first. It’s easier to set up and faster.
-
play_tone
(volume=10, frequency=440, duration=1)[source] Play a tone on the EV3 brick.
- Parameters
volume (int) – The volume of the played tone:
0
to100
frequency (int) – The frequency (Hz) of the played tone:
0
to10000
duration (float) – The duration (s) of the played tone:
0
to5
>>> myev3.play_tone(volume=5, frequency=880, duration=0.5)
-
set_statuslight
(mode='solid', color='green')[source] Set the status light of the EV3 brick.
- Parameters
mode (str) – The light mode:
'solid'
,'pulsing'
,'off'
color (str) – The light color:
'green'
,'orange'
,'red'
>>> myev3.set_statuslight(mode='pulsing', color='orange')
-
property
batterylevel
Contains the EV3 battery level in % (read only).
-
property
connectedsensors
Contains a list with the sensors connected to the EV3 (read only).
-
property
devmode
Contains a developer mode flag. Default is
False
. WhenTrue
, communication info is displayed after each direct command (read/write).