LabJackU3
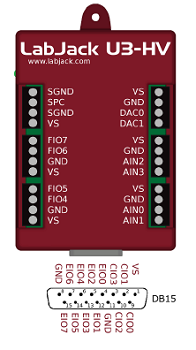
-
class
devices.
LabJackU3
(serialnum=None)[source] The class to represent the LabJack U3.
- Parameters
serialnum (int) – The device serial number.
Ports that are made available with this class are listed below. The port names assume a U3-HV.
Analog Output:
'DAC0'
,'DAC1'
Analog Input:
'AIN0'
,'AIN1'
,'AIN2'
,'AIN3'
Flexible I/O:
'FIO4'
,'FIO5'
,'FIO6'
,'FIO7'
,Flexible I/O:
'EIO0'
,'EIO1'
, … ,'EIO7'
Device-specific methods:
get_config - Gets port configuration
reset_config - Resets port configuration to factory defaults
get_bitdir - Gets digital port bit direction
Connect to the first found U3:
>>> from labjack_unified.devices import LabJackU3 >>> lju3 = LabJackU3() >>> lju3.display_info() >>> lju3.close()
You can also connect to a specific device using its serial number:
>>> lju3 = LabJackU3(320012345)
General
LabJackU3.
get_labjacktemp
(unit='C')[source]Get ambient temperature from LabJack’s internal sensor.
- Parameters
unit (str) – The temperature measurement unit. Valid values are
'C'
or'F'
. Default unit is'C'
.- Returns
The internal sensor temperature reading.
- Return type
float
Get temperature reading in Celsius:
>>> lju3.get_labjacktemp()Get temperature reading in Fahrenheit:
>>> lju3.get_labjacktemp(unit='F')
I/O
LabJackU3.
set_config
(name, config)[source]Set the LabJack flexible IO port configuration.
- Parameters
name (str) – The port name to configure.
config (str, int) – The configuration option. If name is
'ALL'
a string of mask bits for all 16 ports is used. For single port, config can be either a string'analog'
or'digital'
. Alternativelly, 1 or 0 can be used.Configure flexible ports
'FIO4'
and'FIO5'
as analog:>>> lju3.set_config('FIO4', 'analog') >>> lju3.set_config('FIO5', 1)Configure flexible ports
'EIO0'
and'EIO1'
as digital:>>> lju3.set_config('EIO0', 'digital') >>> lju3.set_config('EIO1', 0)Configure flexible ports
'EI01'
and'EI03'
as analog and ports'AIN0'
to'AIN3'
to analog (if a U3-LV is used):>>> lju3.set_config('ALL', '0000101000001111')Note
LSB (Least Significant Bit) is port
'AIN0'
.On a U3-HV, the first 4 ports are always analog and the first 4 LSB settings are ignored.
LabJackU3.
get_config
(name='ALL')[source]Get the LabJack flexible IO port configuration.
- Parameters
name (str) – The port name to get the configuration. If name is
'ALL'
a dictionary with keys EIOAnalog and FIOAnalog is returned.- Returns
For single port name, an integer is returned, where 1=Analog and 0=Digital. If name is
'ALL'
a dictionary containing the bit mask string for the EIO and FIO ports is returned. The least significant bit (LSB) of the EIO and FIO bit strings correspond respectively to ports'EIO0'
and'FIO0'
.- Return type
int, dict
Get flexible port configuration:
>>> lju3.get_config()Note
In the case of a LabJack U3-HV, the four LSBs of the FIO ports are always 1s. They correspond to the always analog ports
'AIN0'
to'AIN3'
.
LabJackU3.
reset_config
()[source]Reset the flexible IO ports to default all digital.
>>> lju3.reset_config()Note
On the LabJack U3-HV the first 4 ports
'AIN0'
to'AIN3'
are always analog.
LabJackU3.
set_digital
(name, state)[source]Write the digital state to an output port. It also sets the port direction to output.
- Parameters
name (str) – The port name to set the state.
state (int) – The digital state 0 = Low, 1 = High.
Set port
'FIO4'
output to high and port'FIO5'
to low:>>> lju3.set_digital('FIO4', 1) >>> lju3.set_digital('FIO5', 0)
LabJackU3.
get_digital
(name)[source]Read the digital state from an input port. It also sets the port direction to input.
- Parameters
name (str) – The port name to get the state.
- Returns
The state of the digital port. 0 = Low, 1 = High.
- Return type
int
Get port
'FIO6'
input state:>>> lju3.get_digital('FIO6')
LabJackU3.
get_bitdir
(name)[source]Read the direction of the digital port.
- Parameters
name (str) – The port name to get the direction.
- Returns
The direction of the digital port. Input or Output.
- Return type
str
Get the direction of port
'FIO6'
:>>> lju3.get_bitdir('FIO6')
LabJackU3.
set_analog
(name, value)[source]Set analog output voltage.
- Parameters
name (str) – The port name to set the output voltage. Available ports are
'DAC0'
and'DAC1'
.value (float) – The output voltage between
0
and5
V.Set port
'DAC1'
output voltage to2.2
V:>>> lju3.set_analog('DAC1', 2.2)
LabJackU3.
get_analog
(namepos, *args)[source]Get analog input voltage.
- Parameters
name (str) – The positive port name to get the voltage.
args (str) –
Can be one of the three options:
'single-ended'
(default value)The negative port name to get a differential voltage.
'special'
to get increased range on the voltage reading.- Returns
The input voltage value.
On a U3-HV, the range for ports
'AIN0'
to'AIN3'
is +/-10 VOn a U3-HV,
'special'
enables a range of -10 to +20 VFIO ports have a range of +/- 2.4 V
FIO ports using
'special'
have a range of 0 to 3.6 V- Return type
float
Get single-ended voltage on port
'FIO2'
:>>> lju3.get_analog('FIO2')Get differential voltage betweens ports
'AIN0'
and'AIN1'
:>>> lju3.get_analog('AIN0', 'AIN1')Get special range voltage on port
'FIO3'
:>>> lju3.get_analog('FIO3', 'special')
Streaming
LabJackU3.
set_stream
(names, scanrate=50000, readrate=0.5)[source]Set and start data streaming.
- Parameters
name (str, list(str)) – The port name (or list of names) to be streamed.
scanrate (int) – The scan rate (Hz) of the data streaming. The default (and maximum) value is
50000
Hz. The effective scan frequency of each port is the scan rate divided by the number of scanned ports.readrate (float) – The rate in seconds at which blocks of data are retrieved from the data buffer. The default value is
0.5
seconds.Set data streaming on port
'AIN0'
at25000
Hz, every0.5
s:>>> lju3.set_stream('AIN0', scanrate=25000, readrate=0.5)Set data streaming on ports
'AIN0'
and'AIN1'
at50000
Hz, every1.0
s:>>> lju3.set_stream(['AIN0', 'AIN1'], scanrate=50000, readrate=1.0)Note
Only analog input ports
'AIN0'
to'AIN3'
can be streamed. Hence, a Labjack U3-HV has to be used. While it’s possible to stream digital ports, that hasn’t been implemented in this release.
LabJackU3.
get_stream
()[source]Get streaming data block.
- Returns
5-tuple
dt
The sampling period (s) between each data point.
data
The numpy m-by-n array containing the streamed data where m is the number of samples per port in the block and n is the number of ports defined in set_stream
numscans
The actual number of scans per port in the data block.
commbacklog
The communication backlog in % (increasing values indicate that the computer cannot keep up with the data download from the U3 driver)
devbacklog
The U3 device backlog in % (increasing values indicate that the device cannot keep up with the data streaming - usually not the case)
- Return type
(float, ndarray, int, float, float)
Retrieve scan period, data, and scan info:
>>> dt, datablock, numscans, commbacklog, U3backlog = lju3.get_stream()
PWM
LabJackU3.
set_pwm
(pwmnum=1, dirport1=None, dirport2=None, frequency=366)[source]Configure PWM output.
- Parameters
pwmnum (int) – The number of PWM output signals.
1
or2
PWMs can be used. For one PWM, the output port is'FIO4'
. For two PWMs, the output ports are'FIO4'
and'FIO5'
.dirport1 (None, str) –
The type of ports that control the PWM direction for electric motor control. There are three options:
None
- Default value (no direction ports are used)
'DAC'
- Uses analog ports'DAC0'
and'DAC1'
'DIO'
- Uses digital ports'FIO6'
and'FIO7'
dirport2 (None, str) – Same as dirport1. It’s used when two PWM outputs are enabled. The
'DAC'
option can only be used for one set of direction ports, unless the two motors are running synchronously. For the'DIO'
option, digital ports'EIO0'
and'EIO1'
are used.frequency (int) – The PWM signal frequency in Hz. In the case of two PWMs, both will have the same frequency. Valid values are
183
,366
or732
.Set 1 PWM for motor control on
'FIO4'
with direction ports on'DAC0'
and'DAC1'
. The PWM frequency is the default366
Hz:>>> lju3.set_pwm(dirport1='DAC')Set 2 PWMs on ports
'FIO4'
and'FIO5'
with a frequency of183
Hz:>>> lju3.set_pwm(pwmnum=2, frequency=183)Set 2 PWMs for motor control on ports
'FIO4'
and'FIO5'
, using the digital ports'FIO6'
and'FIO7'
for motor 1 direction, and'EIO0'
and'EIO1'
for motor 2 direction. The PWM frequency is732
Hz:>>> lju3.set_pwm(pwmnum=2, dirport1='DIO', dirport2='DIO', frequency=732)Note
When using digital ports, a 10 kOhm resistor has to be connected from the LabJack VS port to each one of the DIO ports to ensure true high and low states.
LabJackU3.
set_dutycycle
(value1=None, value2=None, brake1=False, brake2=False)[source]Set PWM duty cycle value.
- Parameters
value1 (float) – The PWM 1 duty cycle percent value between
-100
and100
.value2 (float) – The PWM 2 duty cycle percent value between
-100
and100
.brake1 (bool) – The motor 1 brake option used when dutycycle is zero. Brake is applied when
True
. Motor is floating whenFalse
.brake2 (bool) – The motor 2 brake option used when dutycycle is zero. Brake is applied when
True
. Motor is floating whenFalse
.Set duty cycle to
50
% on PWM 1:>>> lju3.set_dutycycle(value1=50)Set duty cycle to
25
% (reverse rotation) on PWM 2:>>> lju3.set_dutycycle(value2=-25)Set duty cycle to
20
% and40
% on PWMs 1 and 2:>>> lju3.set_dutycycle(value1=20, value2=40)Stop motor 2 and apply brake:
>>> lju3.set_dutycycle(value2=0, brake2=True)Note
Avoid suddenly switching the direction of rotation to avoid damaging the motor.
You can use the brake option True to hold the motor in position.
Quadrature
LabJackU3.
set_quadrature
(zphase1=False)[source]Configure quadrature encoder input on ports
'FIO4'
and'FIO5'
.
- Parameters
zphase1 (bool) – The logic value indicating if a Z phase reference pulse is used on port
'FIO6'
.Set ports
'FIO4'
and'FIO5'
for encoder phase A and B signals:>>> lju3.set_quadrature()Set ports
'FIO4'
and'FIO5'
for encoder phase A and B signals and port'FIO6'
for the reference Z phase:>>> lju3.set_quadrature(zphase1=True)